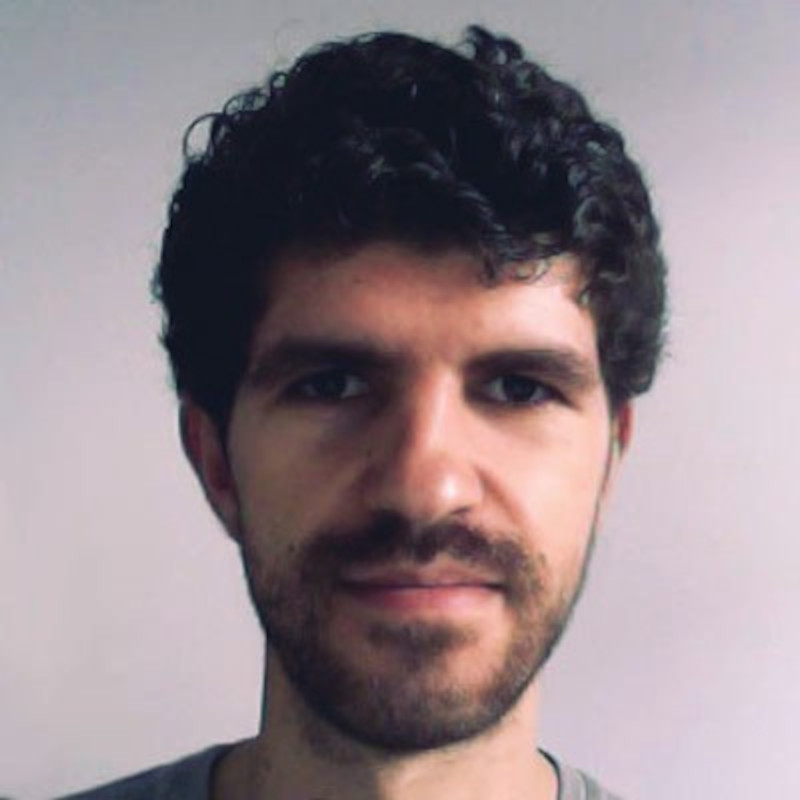
Hi! I’m Nuno Donato 👋
A chronic developer born and living in Portugal.
I started coding with QBasic when I was 7; since then, it has been part of my life. I’ve made simple apps, complex apps, full-stack development on the web (Laravel ❤), designed databases, developed games, and love to imagine and create small tools.
I’m currently working as a senior back-end developer at Trippz.
Ever since I got in touch with the early versions of GPT I became fascinated with the potential of generative AI in our lives and work. Since then I’ve been conducting research and experiments to push the boundaries further.
This website also hosts my main blog where I post everything from tech and parenting to books and simple living.
The best way to reach out to me is via email, but you can also find me on LinkedIn.